Open Space Analysis
I was curious to work with public open space data because of the work of the Milton School Building Committee.
In recent public meetings, there has been much discussion of suitable sites in Milton for the construction of a new school. Because an RFP for the purchase of a suitable land parcel received no responses, the committee focused on currently open land owned by the Town of Milton, restricting its searches to parcels of 6 acres or more. It should be possible to identify all such parcels using the public open space dataset.
Additionally, Milton is famous for its extensive forests and trails, being nestled within the Blue Hills reservation. I wanted to see if this qualitative feature of life in Milton could be shown quantitatively in the open space data. To achieve this, I compare the average distance to the nearest open space parcel for residential land parcels in Milton vs Quincy, our significantly larger and more urban neighbor. I wanted to see if the average residential parcel is closer to open space in Milton than in Quincy.
[1]:
import geopandas as gpd
import folium
import joblib
import matplotlib.pyplot as plt
import seaborn as sns
from IPython.display import Markdown, display
import milton_maps as mm
sns.set_style("darkgrid", {"axes.facecolor": ".9"})
sns.set(rc = {'figure.figsize':(15,8)})
sns.set_context("notebook")
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/geopandas/_compat.py:123: UserWarning: The Shapely GEOS version (3.10.3-CAPI-1.16.1) is incompatible with the GEOS version PyGEOS was compiled with (3.10.4-CAPI-1.16.2). Conversions between both will be slow.
warnings.warn(
Load cleaned and processed input files
This notebook works from cleaned and transformed data. The transformation pipeline is defined in dvc.yaml
. To simply work with the latest version, pull the appropriate versions from dvc using dvc pull
. To regenerate the files, perhaps with local code changes, run dvc repro
.
[2]:
town_boundaries = gpd.read_file("../data/processed/town_boundaries.shp.zip").set_index("TOWN_ID")
milton_quincy_boundaries = town_boundaries[town_boundaries.TOWN.isin(["MILTON", "QUINCY"])]
milton_quincy_boundaries.shape
[2]:
(2, 19)
[3]:
openspace = gpd.read_file("../data/processed/openspace.shp.zip", mask=milton_quincy_boundaries)
openspace.shape
[3]:
(767, 62)
[4]:
residential_tax_parcels = joblib.load("../data/processed/residential_tax_parcels.pkl")
residential_tax_parcels.shape
[4]:
(32387, 28)
Data review
In order to contextualize the open space data maps, we’ll plot them on top of the town boundary shapes for Milton and Quincy. Load the town boundary data:
[5]:
fig, ax = plt.subplots(1, figsize=(10,10))
mm.plot_map(milton_quincy_boundaries,
column="TOWN",
cmap="Pastel2",
title="Milton and Quincy",
legend=False,
ax=ax)
for i in milton_quincy_boundaries.index:
x = town_boundaries.loc[[i]].representative_point().x
y = town_boundaries.loc[[i]].representative_point().y
plt.annotate(town_boundaries.loc[i, "TOWN"], (x,y))
plt.show()
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:1475: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
x = float(self.convert_xunits(x))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:1477: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
y = float(self.convert_yunits(y))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:757: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
posx = float(self.convert_xunits(self._x))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:758: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
posy = float(self.convert_yunits(self._y))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:897: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
x = float(self.convert_xunits(self._x))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:898: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
y = float(self.convert_yunits(self._y))
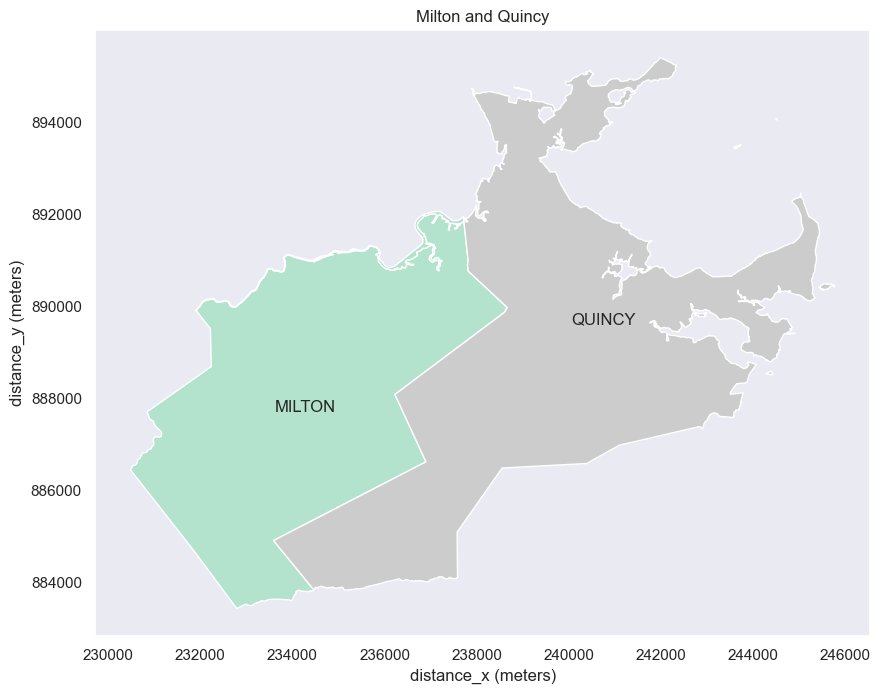
Open space maps
Let’s get a quick visualization of open spaces on our map of Milton and Quincy. The following plot color codes the open spaces by the name of its Manager.
[6]:
mm.plot_map(openspace,
column="MANAGER",
ax=ax,
legend_shift=1.8,
title="Milton and Quincy Open Spaces",
alpha=0.5)
display(fig)
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:1475: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
x = float(self.convert_xunits(x))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:1477: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
y = float(self.convert_yunits(y))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:757: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
posx = float(self.convert_xunits(self._x))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:758: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
posy = float(self.convert_yunits(self._y))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:897: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
x = float(self.convert_xunits(self._x))
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/matplotlib/text.py:898: FutureWarning: Calling float on a single element Series is deprecated and will raise a TypeError in the future. Use float(ser.iloc[0]) instead
y = float(self.convert_yunits(self._y))
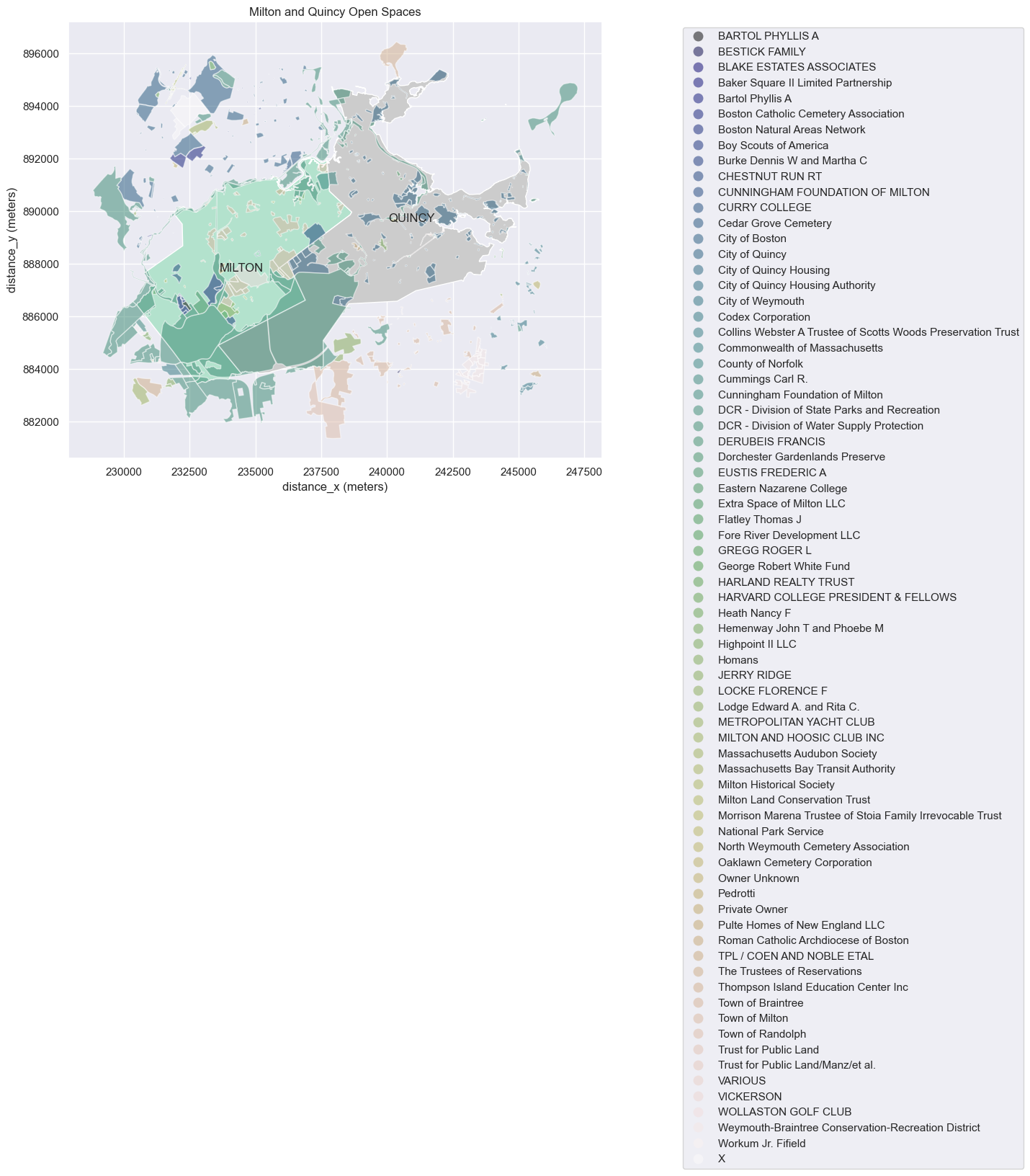
<Figure size 1500x800 with 0 Axes>
Now let’s use the folium
package to plot this data on top of an interactive street map.
[7]:
map_center = milton_quincy_boundaries.dissolve().centroid.to_crs(epsg=4326)[0]
[29]:
milton_map = folium.Map(width=800,height=800, location=[map_center.y, map_center.x], zoom_start=12)
sf, cm_dict = mm.make_choropleth_style_function(openspace, "PUB_ACCESS", "Dark2")
#Print a legend
display(Markdown("""**Explorable Map of Open Space**
You can pan and zoom the map below to explore open space in Quincy and Milton.
Click on open space parcels to see a pop-up with more information.
""" + mm.html_legend(cm_dict)))
folium.GeoJson(milton_quincy_boundaries.to_crs("EPSG:3857").geometry).add_to(milton_map)
# We need to convert the openspace geodataframe to the EPSG:3857 coordinate reference system (CRS) because that is the default for folium maps.
folium.GeoJson(data=openspace.to_crs("EPSG:3857"),
style_function=sf,
popup=folium.GeoJsonPopup(
fields=['SITE_NAME', 'MANAGER', 'GIS_ACRES', "PRIM_PURP", "PUB_ACCESS", "LEV_PROT"],
aliases=['Name','Managed by','Acres', "Primary Purpose", "Public Access", "Level of Protection"]
)).add_to(milton_map)
display(milton_map)
Explorable Map of Open Space
You can pan and zoom the map below to explore open space in Quincy and Milton. Click on open space parcels to see a pop-up with more information.
Value | Color |
---|---|
Yes (open to public) | ████████ |
Limited (membership only) | ████████ |
Unknown | ████████ |
No (not open to public) | ████████ |
Calculate distribution of distance to openspace for residential parcels
Finally, let’s tackle our question of whether Quincy residents are further from open space on average compared to Milton residents.
Let’s first filter the open space parcels down to those that are publicly accessible:
[9]:
openspace['PUB_ACCESS'].unique()
[9]:
array(['Yes (open to public)', 'Limited (membership only)', 'Unknown',
'No (not open to public)'], dtype=object)
[10]:
accessible_openspace = openspace[openspace['PUB_ACCESS']=="Yes (open to public)"]
The following geopandas spatial join links each residential land parcel to the nearest open space land parcel, using the closest distance between the two shapes. It can produce multiple output rows per residential land parcel if there are multiple open space parcels that are equidistant with the shortest distance.
[11]:
result = gpd.sjoin_nearest(residential_tax_parcels, accessible_openspace, how="left", distance_col="distance_to_openspace")
result.head()
[11]:
SHAPE_Leng | SHAPE_Area | MAP_PAR_ID | POLY_TYPE | MAP_NO | SOURCE | PLAN_ID | LAST_EDIT | BND_CHK | NO_MATCH | ... | INTSYM | OS_ID | CAL_DATE_R | FORMAL_SIT | CR_REF | OS_TYPE | EEA_CR_ID | SHAPE_AREA | SHAPE_LEN | distance_to_openspace | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
LOC_ID | |||||||||||||||||||||
189M 2 6 | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | ... | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN |
2436080Q_22_22 | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | ... | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN |
F_757603_2907021 | 718.018773 | 21213.803766 | A 10 1B | FEE | A | ASSESS | A-9-10 | 20180101.0 | NaN | N | ... | NaN | 50-173 | 2019-06-11 | NaN | 0.0 | NaN | NaN | 1350.764294 | 155.163256 | 0.0 |
F_757603_2907021 | 718.018773 | 21213.803766 | A 10 1B | FEE | A | ASSESS | A-9-10 | 20180101.0 | NaN | N | ... | NaN | 189-91 | 2019-06-11 | NaN | 0.0 | NaN | NaN | 1218.553710 | 145.826404 | 0.0 |
F_757603_2907021 | 718.018773 | 21213.803766 | A 10 1B | FEE | A | ASSESS | A-9-10 | 20180101.0 | NaN | N | ... | NaN | 189-92 | 2019-06-11 | NaN | 0.0 | NaN | NaN | 8290.233976 | 487.599831 | 0.0 |
5 rows × 91 columns
Plot the distribution of distance to the nearest open land for Milton and for Quincy.
It looks like Quincy residents are just as close to the nearest open land as their Milton neighbors, on average. That’s a little surprising!
[12]:
g = sns.displot(result.reset_index(), x='distance_to_openspace', hue="TOWN", kind="kde", common_norm=True)
g.set(xlabel="Distance to public openspace (meters)",
ylabel="Number of residential parcels",
title="Distribution of distance to openspace by tax parcel")
plt.show()
/Users/alexhasha/Library/Caches/pypoetry/virtualenvs/milton-maps-gfMaDXEA-py3.10/lib/python3.10/site-packages/seaborn/axisgrid.py:118: UserWarning: The figure layout has changed to tight
self._figure.tight_layout(*args, **kwargs)
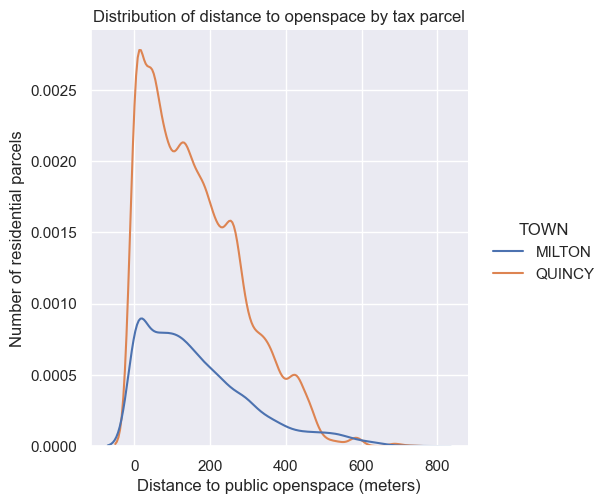
Quantifying htis in a table, we see the median Milton residence is 133 meters from the nearest open space, versus 139 meters for Quincy. Not a big difference!
[13]:
result.groupby("TOWN")["distance_to_openspace"].quantile([0.1,0.25,0.5,0.75,0.9, 1])
[13]:
TOWN
MILTON 0.10 5.712570
0.25 53.907962
0.50 133.377918
0.75 241.847707
0.90 365.795553
1.00 765.278952
QUINCY 0.10 10.835055
0.25 54.520340
0.50 139.640397
0.75 245.533747
0.90 342.402368
1.00 741.994475
Name: distance_to_openspace, dtype: float64
Can we find any quantitative metric that captures that Milton is a “greener” town than Quincy? Yes, if we look at the sizes of the nearest open land, then we see a difference:
[14]:
result.groupby("TOWN")["GIS_ACRES"].quantile([0.1,0.25,0.5,0.75,0.9, 1])
[14]:
TOWN
MILTON 0.10 1.123130
0.25 2.374769
0.50 10.360659
0.75 41.058375
0.90 91.141803
1.00 850.270058
QUINCY 0.10 0.464241
0.25 1.019486
0.50 4.073247
0.75 8.028349
0.90 45.769913
1.00 1753.275872
Name: GIS_ACRES, dtype: float64
While the median Milton residence is no closer to open space than its Quincy counterpart, the median acreage of that open plot is more than twice as large, 10 acres in Milton vs 4 acres in Quincy.
Another random question we can ask of this data: which specific open spaces are the nearest open spaces for the most residence?
[15]:
result.groupby("SITE_NAME").agg(
num_houses=('SITE_NAME', 'count'),
median_distance=('distance_to_openspace', 'median'),
acreage=('GIS_ACRES', 'max'),
).sort_values(by="num_houses", ascending=False)
[15]:
num_houses | median_distance | acreage | |
---|---|---|---|
SITE_NAME | |||
Neponset River Reservation | 1793 | 83.156849 | 109.972767 |
Furnace Brook Parkway | 1377 | 56.429668 | 13.560602 |
Blue Hills Reservation | 1285 | 89.716382 | 1753.275872 |
Quincy Shore Reservation | 1102 | 129.058568 | 70.234147 |
Mass Field Playground | 1051 | 273.067339 | 0.860044 |
... | ... | ... | ... |
Beechwood Knoll School Playground | 11 | 43.317348 | 0.198522 |
Jean Kennedy Playground | 9 | 112.574221 | 0.158380 |
Lamb Conservation Area | 5 | 234.870044 | 7.621469 |
Brooks Field | 3 | 58.219847 | 14.741131 |
Front - Heron Beach | 2 | 38.887931 | 0.380590 |
132 rows × 3 columns
School Building candidate parcels
The school building committee was looking at open spaces owned by the Town of Milton of at least 6 acres. Can we see how many of these there are and where they’re located?
[16]:
openspace.loc[openspace.FEE_OWNER.str.lower().str.contains("milton"), "FEE_OWNER"].unique()
[16]:
array(['Town of Milton', 'Cunningham Foundation of Milton',
'Milton Historical Society', 'CUNNINGHAM FOUNDATION OF MILTON',
'Extra Space of Milton LLC', 'MILTON AND HOOSIC CLUB INC',
'Milton Land Conservation Trust'], dtype=object)
[17]:
school_site_candidates = openspace[(openspace.FEE_OWNER=="Town of Milton") & (openspace.GIS_ACRES >= 6.0)]
school_site_candidates
[17]:
TOWN_ID | POLY_ID | SITE_NAME | FEE_OWNER | OWNER_ABRV | OWNER_TYPE | MANAGER | MANAGR_ABR | MANAGR_TYP | PRIM_PURP | ... | INTSYM | OS_ID | CAL_DATE_R | FORMAL_SIT | CR_REF | OS_TYPE | EEA_CR_ID | SHAPE_AREA | SHAPE_LEN | geometry | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
100 | 189 | 3058 | Milton High School Athletic Fields | Town of Milton | M189 | M | Town of Milton | M189SD | M | Recreation (activities are facility based) | ... | NaN | 189-3058 | NaN | NaN | 0 | NaN | NaN | 57890.235319 | 971.136985 | POLYGON ((234089.437 889234.177, 234044.386 88... |
101 | 189 | 58 | Lamb Conservation Area | Town of Milton | M189 | M | Town of Milton | M189CC | M | Conservation (activities are non-facility based) | ... | NaN | 189-58 | 1978-12-15 | NaN | 0 | NaN | NaN | 30842.988911 | 1239.561577 | POLYGON ((233571.280 889147.018, 233560.965 88... |
103 | 189 | 3131 | Brooks Field | Town of Milton | M189 | M | Town of Milton | M189CC | M | Recreation (activities are facility based) | ... | NaN | 189-3131 | 1978-12-15 | NaN | 0 | NaN | NaN | 59655.241145 | 1196.681131 | POLYGON ((233866.667 888929.914, 233847.029 88... |
107 | 189 | 3064 | Turners Pond Park | Town of Milton | M189 | M | Town of Milton | M189PD | M | Recreation (activities are facility based) | ... | NaN | 189-3064 | NaN | NaN | 0 | NaN | NaN | 64841.955138 | 2770.344170 | POLYGON ((234863.391 890423.323, 234878.905 89... |
116 | 189 | 3132 | Pine Tree Brook Flood Control Area | Town of Milton | M189 | M | Town of Milton | NaN | NaN | Flood Control | ... | NaN | 189-3132 | 1970-01-26 | NaN | 0 | NaN | NaN | 39240.334627 | 794.118748 | POLYGON ((234576.242 886886.138, 234485.339 88... |
136 | 189 | 3046 | Milton Town Forest | Town of Milton | M189 | M | Town of Milton | NaN | NaN | Other (explain) | ... | NaN | 189-3046 | NaN | NaN | 0 | NaN | NaN | 196125.799282 | 2696.309736 | POLYGON ((234128.369 886576.782, 234091.657 88... |
137 | 189 | 3121 | Milton Town Forest | Town of Milton | M189 | M | Town of Milton | NaN | NaN | Other (explain) | ... | NaN | 189-3121 | NaN | NaN | 0 | NaN | NaN | 97320.854000 | 1776.836867 | POLYGON ((234483.783 887004.618, 234485.339 88... |
252 | 189 | 55 | Popes Pond | Town of Milton | M189 | M | Town of Milton | M189CC | M | Recreation and Conservation | ... | NaN | 189-55 | NaN | NaN | 0 | NaN | NaN | 166157.348118 | 2971.820610 | POLYGON ((233516.744 889267.755, 233380.075 88... |
276 | 189 | 62 | Granite Links Golf Course | Town of Milton | M189 | M | Town of Milton | NaN | P | Recreation (activities are facility based) | ... | NaN | 189-62 | NaN | NaN | 0 | NaN | NaN | 166980.724412 | 1786.501274 | POLYGON ((236385.759 887457.414, 236325.733 88... |
281 | 189 | 60 | Granite Links Golf Course | Town of Milton | M189 | M | Town of Milton | NaN | P | Recreation (activities are facility based) | ... | NaN | 189-60 | NaN | NaN | 0 | NaN | NaN | 391054.147570 | 3968.880537 | POLYGON ((236251.722 888445.178, 236268.549 88... |
302 | 189 | 51 | John L. Kelly Athletic Field | Town of Milton | M189 | M | Town of Milton | M189PD | M | Recreation (activities are facility based) | ... | NaN | 189-51 | NaN | NaN | 0 | NaN | NaN | 42567.251734 | 985.612844 | POLYGON ((234246.070 889966.773, 234203.924 88... |
339 | 189 | 3049 | NaN | Town of Milton | M189 | M | Town of Milton | M189CC | M | Conservation (activities are non-facility based) | ... | NaN | 189-3049 | NaN | NaN | 0 | NaN | NaN | 121435.137295 | 1990.220341 | POLYGON ((235895.219 887833.784, 235848.785 88... |
375 | 189 | 3084 | Andrews Park | Town of Milton | M189 | M | Town of Milton | M189PD | M | Recreation (activities are facility based) | ... | NaN | 189-3084 | NaN | NaN | 0 | NaN | NaN | 36491.121776 | 983.305809 | POLYGON ((238324.629 890145.620, 238318.139 89... |
414 | 189 | 3047 | Pine Tree Brook Flood Control Area | Town of Milton | M189 | M | Town of Milton | NaN | NaN | Flood Control | ... | NaN | 189-3047 | 1969-12-05 | NaN | 0 | NaN | NaN | 198081.967686 | 2431.388922 | POLYGON ((234762.419 887207.259, 234659.468 88... |
472 | 189 | 3123 | Arrowhead Lane Conservation Area | Town of Milton | M189 | M | Town of Milton | M189CC | M | Conservation (activities are non-facility based) | ... | NaN | 189-3123 | 1988-09-28 | NaN | 0 | NaN | NaN | 42717.050209 | 901.536225 | POLYGON ((234338.995 887342.436, 234353.529 88... |
496 | 189 | 3080 | Collicot School & Mary A Cunningham | Town of Milton | M189 | M | Town of Milton | M189SD | M | Recreation (activities are facility based) | ... | NaN | 189-3080 | NaN | NaN | 0 | NaN | NaN | 43823.100850 | 1099.628252 | POLYGON ((237005.704 889367.751, 236909.454 88... |
501 | 189 | 3116 | Day | Town of Milton | M189 | M | Town of Milton | NaN | NaN | Conservation (activities are non-facility based) | ... | NaN | 189-3116 | 1965-11-03 | NaN | 0 | NaN | NaN | 79315.815946 | 1681.714169 | POLYGON ((237722.071 891846.733, 237730.959 89... |
618 | 189 | 3073 | Milton Cemetery | Town of Milton | M189 | M | Town of Milton | NaN | NaN | Recreation (activities are facility based) | ... | NaN | 189-3073 | NaN | NaN | 0 | NaN | NaN | 396614.767700 | 4454.225774 | POLYGON ((236379.674 889813.811, 236344.234 88... |
18 rows × 62 columns
[18]:
milton_boundaries = town_boundaries[town_boundaries.TOWN.isin(["MILTON"])]
map_center = milton_boundaries.dissolve().centroid.to_crs(epsg=4326)[0]
[19]:
milton_map = folium.Map(width=800,height=800, location=[map_center.y, map_center.x], zoom_start=13)
sf, cm_dict = mm.make_choropleth_style_function(school_site_candidates, "PRIM_PURP", "Dark2")
#Print a legend
display(Markdown("""**Explorable Map of School Site Candidates**
You can pan and zoom the map below to explore school site candidates in Milton.
Click on open space parcels to see a pop-up with more information.
""" + mm.html_legend(cm_dict)))
folium.GeoJson(milton_boundaries.to_crs("EPSG:3857").geometry).add_to(milton_map)
# We need to convert the openspace geodataframe to the EPSG:3857 coordinate reference system (CRS) because that is the default for folium maps.
folium.GeoJson(data=school_site_candidates.to_crs("EPSG:3857"),
style_function=sf,
popup=folium.GeoJsonPopup(
fields=['SITE_NAME', 'GIS_ACRES', "PRIM_PURP", "PUB_ACCESS", "LEV_PROT", "COMMENTS"],
aliases=['Name','Managed by','Acres', "Primary Purpose", "Public Access", "Level of Protection"]
)).add_to(milton_map)
display(milton_map)
Explorable Map of School Site Candidates
You can pan and zoom the map below to explore school site candidates in Milton. Click on open space parcels to see a pop-up with more information.
Value | Color |
---|---|
Recreation (activities are facility based) | ████████ |
Conservation (activities are non-facility based) | ████████ |
Flood Control | ████████ |
Other (explain) | ████████ |
Recreation and Conservation | ████████ |
We can see that many town-owned parcels of the right size have existing recreational facilities or have a flood-control purpose and hence are not suitable for building. The orange parcels are the remaining sites, and we can see that one of the remaining three is the much discussed Lamb Conservation Area.
How much openspace does Milton have? How much is in conservation status?
[20]:
milton_openspace = openspace.loc[openspace.TOWN_ID==189]
milton_openspace.head()
[20]:
TOWN_ID | POLY_ID | SITE_NAME | FEE_OWNER | OWNER_ABRV | OWNER_TYPE | MANAGER | MANAGR_ABR | MANAGR_TYP | PRIM_PURP | ... | INTSYM | OS_ID | CAL_DATE_R | FORMAL_SIT | CR_REF | OS_TYPE | EEA_CR_ID | SHAPE_AREA | SHAPE_LEN | geometry | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
100 | 189 | 3058 | Milton High School Athletic Fields | Town of Milton | M189 | M | Town of Milton | M189SD | M | Recreation (activities are facility based) | ... | NaN | 189-3058 | NaN | NaN | 0 | NaN | NaN | 57890.235319 | 971.136985 | POLYGON ((234089.437 889234.177, 234044.386 88... |
101 | 189 | 58 | Lamb Conservation Area | Town of Milton | M189 | M | Town of Milton | M189CC | M | Conservation (activities are non-facility based) | ... | NaN | 189-58 | 1978-12-15 | NaN | 0 | NaN | NaN | 30842.988911 | 1239.561577 | POLYGON ((233571.280 889147.018, 233560.965 88... |
102 | 189 | 3130 | Pierce Middle School Athletic Fields | Town of Milton | M189 | M | Town of Milton | M189SD | M | Recreation (activities are facility based) | ... | NaN | 189-3130 | NaN | NaN | 0 | NaN | NaN | 9610.348045 | 453.437248 | POLYGON ((234292.207 889748.966, 234286.572 88... |
103 | 189 | 3131 | Brooks Field | Town of Milton | M189 | M | Town of Milton | M189CC | M | Recreation (activities are facility based) | ... | NaN | 189-3131 | 1978-12-15 | NaN | 0 | NaN | NaN | 59655.241145 | 1196.681131 | POLYGON ((233866.667 888929.914, 233847.029 88... |
104 | 189 | 3059 | Pine Tree Brook Greenway | Owner Unknown | NaN | X | Owner Unknown | NaN | NaN | Recreation (activities are facility based) | ... | NaN | 189-3059 | NaN | NaN | 0 | NaN | NaN | 4865.273125 | 1608.666884 | POLYGON ((233552.836 889220.870, 233552.322 88... |
5 rows × 62 columns
[21]:
openspace.TOWN_ID.unique()
[21]:
array([243, 189, 336, 40, 35, 244, 50, 142, 73])
[22]:
milton_openspace.PRIM_PURP.value_counts()
[22]:
PRIM_PURP
Recreation and Conservation 47
Conservation (activities are non-facility based) 44
Recreation (activities are facility based) 31
Other (explain) 7
Historical/Cultural 7
Agricultural 6
Flood Control 2
Name: count, dtype: int64
[23]:
milton_map = folium.Map(width=800,height=800, location=[map_center.y, map_center.x], zoom_start=12)
sf, cm_dict = mm.make_choropleth_style_function(openspace, "PRIM_PURP", "Dark2")
#Print a legend
display(Markdown("""**Explorable Map of Open Space**
You can pan and zoom the map below to explore open space in Quincy and Milton.
Click on open space parcels to see a pop-up with more information.
""" + mm.html_legend(cm_dict)))
folium.GeoJson(milton_quincy_boundaries.to_crs("EPSG:3857").geometry).add_to(milton_map)
# We need to convert the openspace geodataframe to the EPSG:3857 coordinate reference system (CRS) because that is the default for folium maps.
folium.GeoJson(data=milton_openspace.to_crs("EPSG:3857"),
style_function=sf,
popup=folium.GeoJsonPopup(
fields=['SITE_NAME', 'MANAGER', 'GIS_ACRES', "PRIM_PURP", "PUB_ACCESS", "LEV_PROT"],
aliases=['Name','Managed by','Acres', "Primary Purpose", "Public Access", "Level of Protection"]
)).add_to(milton_map)
display(milton_map)
Explorable Map of Open Space
You can pan and zoom the map below to explore open space in Quincy and Milton. Click on open space parcels to see a pop-up with more information.
Value | Color |
---|---|
Recreation (activities are facility based) | ████████ |
Conservation (activities are non-facility based) | ████████ |
Flood Control | ████████ |
Historical/Cultural | ████████ |
Water Supply Protection | ████████ |
Site is underwater | ████████ |
Recreation and Conservation | ████████ |
Other (explain) | ████████ |
Agricultural | ████████ |
Unknown | ████████ |
[24]:
conservation_land_purposes = [
'Conservation (activities are non-facility based)',
'Recreation and Conservation',
]
conservation_area = milton_openspace.loc[milton_openspace.PRIM_PURP.isin(conservation_land_purposes), "SHAPE_AREA"].sum()
[25]:
conservation_area
[25]:
10017849.02254728
[26]:
milton_openspace.crs
[26]:
<Projected CRS: EPSG:26986>
Name: NAD83 / Massachusetts Mainland
Axis Info [cartesian]:
- X[east]: Easting (metre)
- Y[north]: Northing (metre)
Area of Use:
- name: United States (USA) - Massachusetts onshore - counties of Barnstable; Berkshire; Bristol; Essex; Franklin; Hampden; Hampshire; Middlesex; Norfolk; Plymouth; Suffolk; Worcester.
- bounds: (-73.5, 41.46, -69.86, 42.89)
Coordinate Operation:
- name: SPCS83 Massachusetts Mainland zone (meters)
- method: Lambert Conic Conformal (2SP)
Datum: North American Datum 1983
- Ellipsoid: GRS 1980
- Prime Meridian: Greenwich
[27]:
SQ_METERS_PER_ACRE = 4046.86
[28]:
conservation_area / SQ_METERS_PER_ACRE
[28]:
2475.4622157789695
[ ]: